Dart 2.17 Highlights: Enhanced enums, Super initializers support and more
In this article, we outlined what is new in Dart 2.17
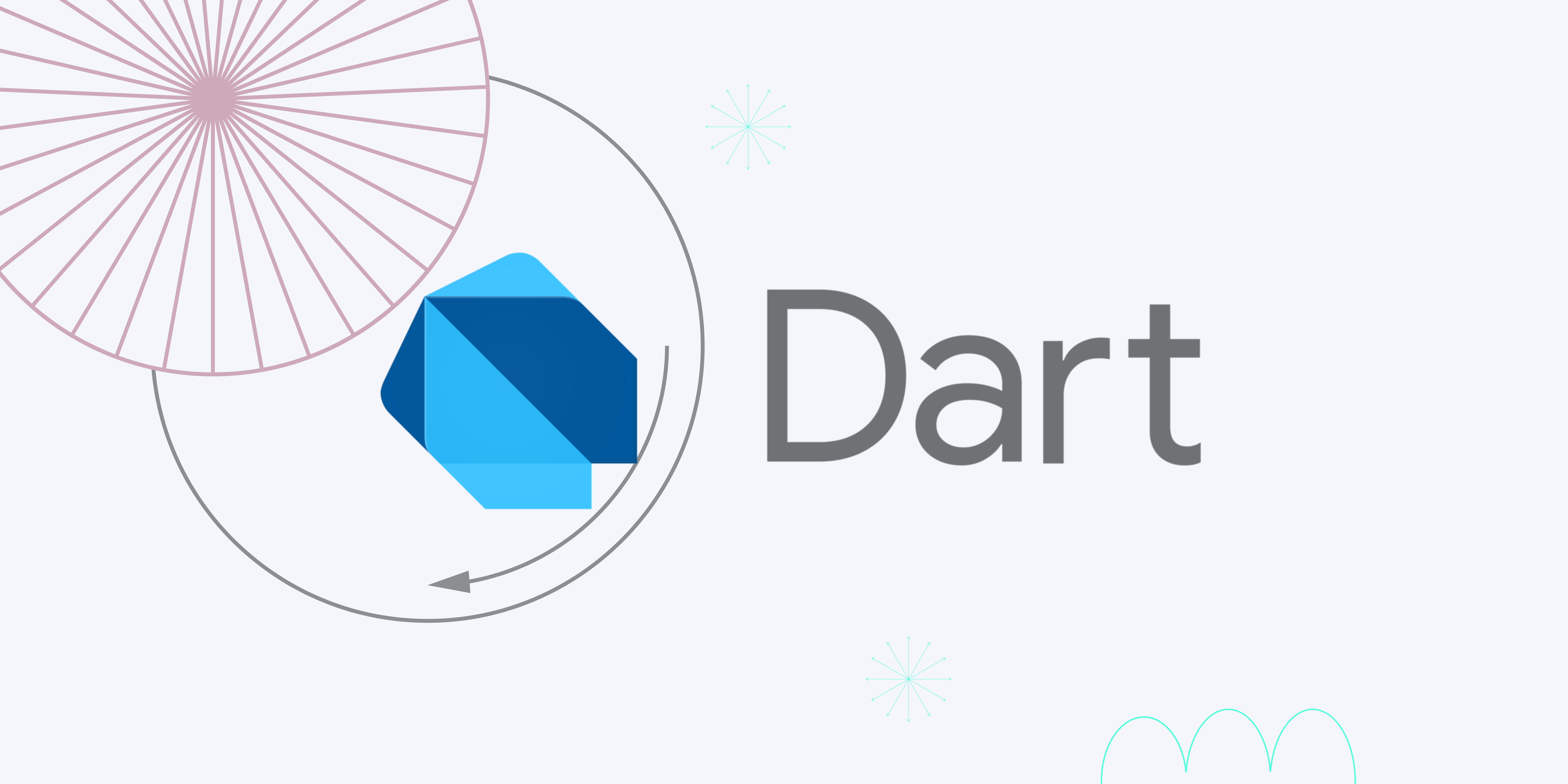
Table of content
In the latest major release of Flutter SDK (version 3.0.0), the Google team introduced many improvements for Desktop app development and overall tooling and performance improvement. Along with Flutter 3, Dart 2.17 was also introduced. The Dart team has put quite an effort into improving productivity for the developers. Now developers can use enhanced enum types, simplified super constructors, named parameter positioning anywhere, and many more. Today we will discuss these changes.
Enhanced enums with members
Enum types of objects are convenient when it comes to defining states of something. For example, we can have a Flower object which can contain different types of flowers. We can say flowers can contain a set of flower names.
For example:
We can have enum type Flower, and it can hold different flowers,
like rose, daisy, sunFlower, etc. Now, if we want to hold the color of each flower and then print out the object that the rose is pink? We could use an extension that can carefully check which flower type has which color by matching with the object and manually doing it. But in Dart 2.17, we can use the enum to add more fields to the object and provide the values through the constructor or even override the members' methods.
We can have a look at the before and after codes to compare the changes:
We can see from the photos that after Dart 2.17, we can add a new field to the enum object’s constructor named color, which contains the Color property of the flower. And then, we can override the toString method to print out the details of the flower. The code looks much simpler and easy to read.
Super initializers support
We all worked with object-oriented programming while working with Flutter. When creating an inherited object, we need to pass two sets of different values, one is for the inherited class, and another is for the superclass's constructor, which increases the code volume by quite a bit. And it becomes hard to read as the code keeps increasing. Now with Dart 2.17, developers can directly pass the parameter to the super constructor from the child class's constructor without invoking the super constructor.
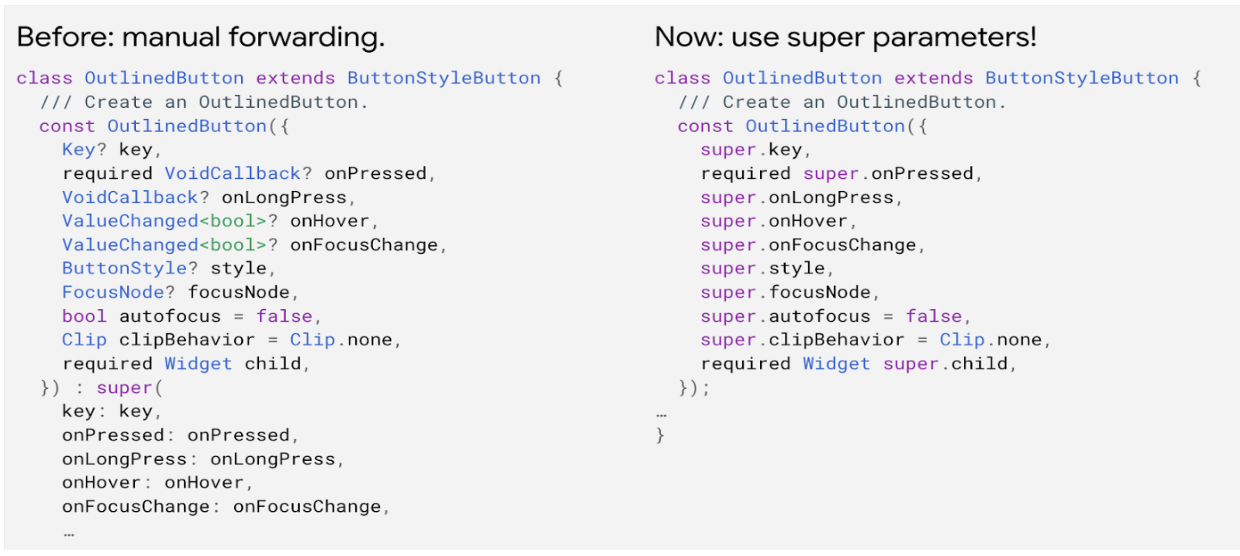
We can see that developers can now directly pass values for super constructor using super parameters, which will reduce a lot of boilerplate code and repetition. The Flutter team was able to reduce almost 2000 lines of code by using super parameters.
Named args in method everywhere
Before Dart 2.17 if we had to use named arguments, we would have to put them at the last while invoking the method. This could be a problem, while sometimes we probably need to put the named arguments first before the positional arguments to make the code more readable. Now Dart 2.17 supports putting the named arguments anywhere while invoking the method.
More sample codes can be found here.
Other updates
The latest Dart SDK has upgraded the lint package to improve code writing practice and keep an eye on the bad patterns. It includes 10 new rules for Dart-lang and also 2 new rules, especially for Flutter. This ensures a consistent coding style for the projects by leveraging the powerful Dart Analyzer.
Dart-lang updated the documentation as well. Different surveys concluded that developers love to read example code along with documentation. And they listened to it and updated documentation with sample codes as well. For example, the dart:convert package now has much more detailed documentation than the 2.16 version.
Other than that, there have been several updates made regarding platform integration. Flutter tools have a new template for developing plugin packages using Dart FFI. dart:ffi lies at the heart of communicating with c/native codes. More details:https://dart.dev/guides/libraries/c-interop
Also, the garbage collector used with the native side of the code is now smarter than before. Now it keeps information on which resources to free or not to free by utilizing a new concept called Finazlier. Also, Dart-lang lets you compile dart code into executables that will not need Dart SDK installed on the machine it is running on.
Conclusion
The dart team is making an effort to make the developers' lives easier by providing a simplified code structure that is more readable and manageable. Also, they are making sure that Dart support reaches as many platforms as possible. Hopefully, our summary of Dart 2.17 will provide some valuable insight into Dart-lang changes and see you soon!