A Complete Guide to Widget Composition in Flutter
Here’s everything you need to know about widget composition in Flutter, from best practices to examples for building a UI. Code like a pro, the Apexive way.
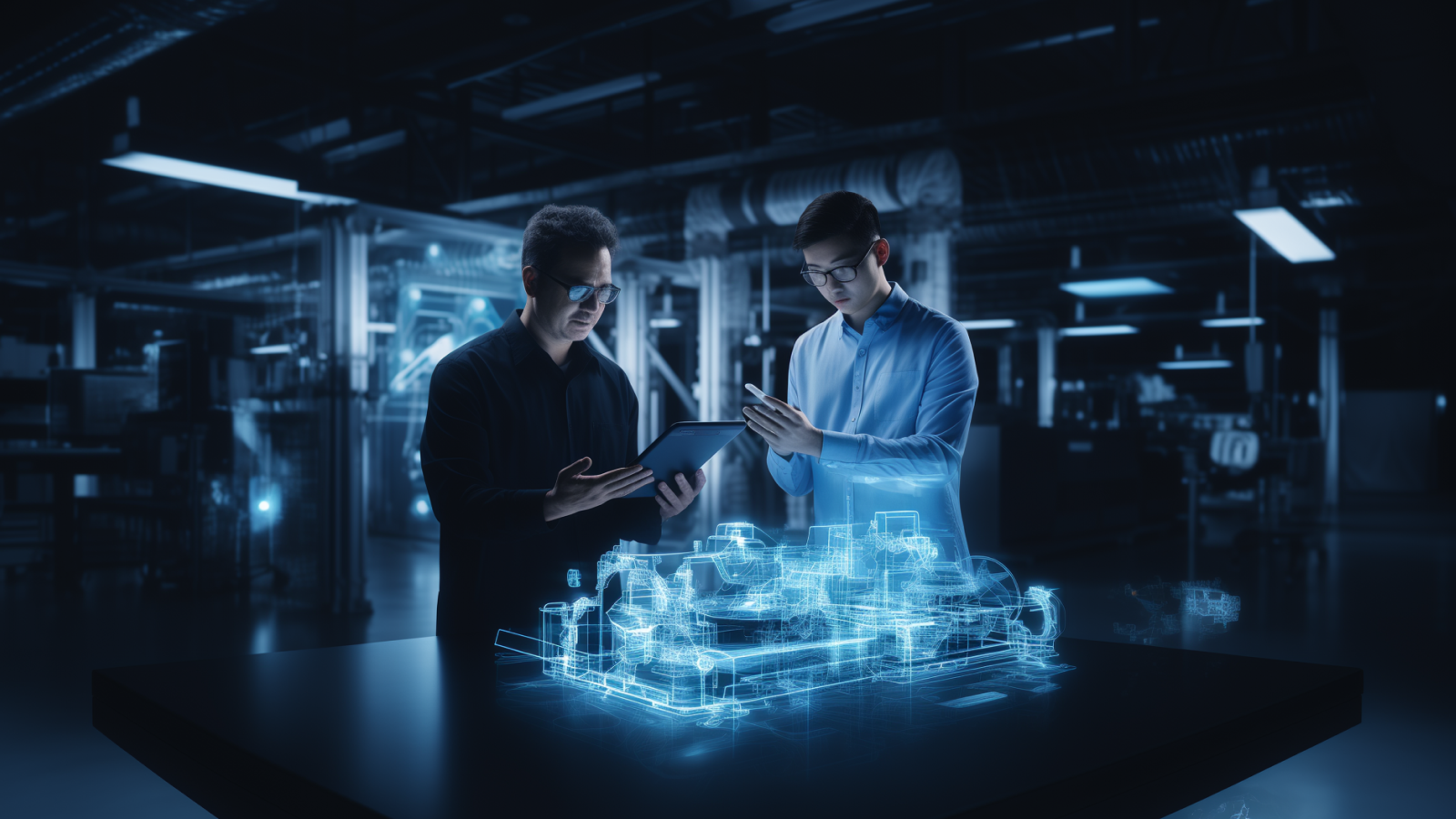
Table of content
Flutter is Google’s famous UI toolkit that lets you build beautiful, native apps for mobile, web, and desktop from a single codebase. So what’s the secret sauce behind crafting stunning apps and visual experiences in Flutter? A concept called widget composition.
Think of widgets as the building blocks of your app's interface. Each widget has a specific purpose or task—like displaying text, showing an image, or adding a button. They're essentially the Lego bricks of app development. But instead of interlocking physically, they nest and combine inside each other within your code.
As a Flutter developer, mastering widget composition isn't just about code. You need to think modularly and creatively. It's about knowing how to combine simple, single-purpose widgets to build more complex, reusable, and effective UI components. Doing this enhances the readability and maintainability of your code. It also aligns perfectly with the declarative nature of Flutter, where you describe what you want your UI to look like and let the framework take care of the rendering.
Let’s dive into the world of widget composition. Whether you're just starting out, or a seasoned developer looking to refine your expertise, this guide will take your Flutter development skills to new heights.
Understanding the Fundamentals of Widget Composition in Flutter
Widgets form the backbone of any application built in Flutter. Think of them as a blueprint for a UI element. Each widget handles a specific task and comes with its own properties you can customize. This could be anything from a simple text label, an image, or a button, to more complex structures like a dialog box. Widgets in Flutter do everything from controlling layout aspects like padding and alignment, to defining the style and behavior of the UI.
Types of Widgets in Flutter
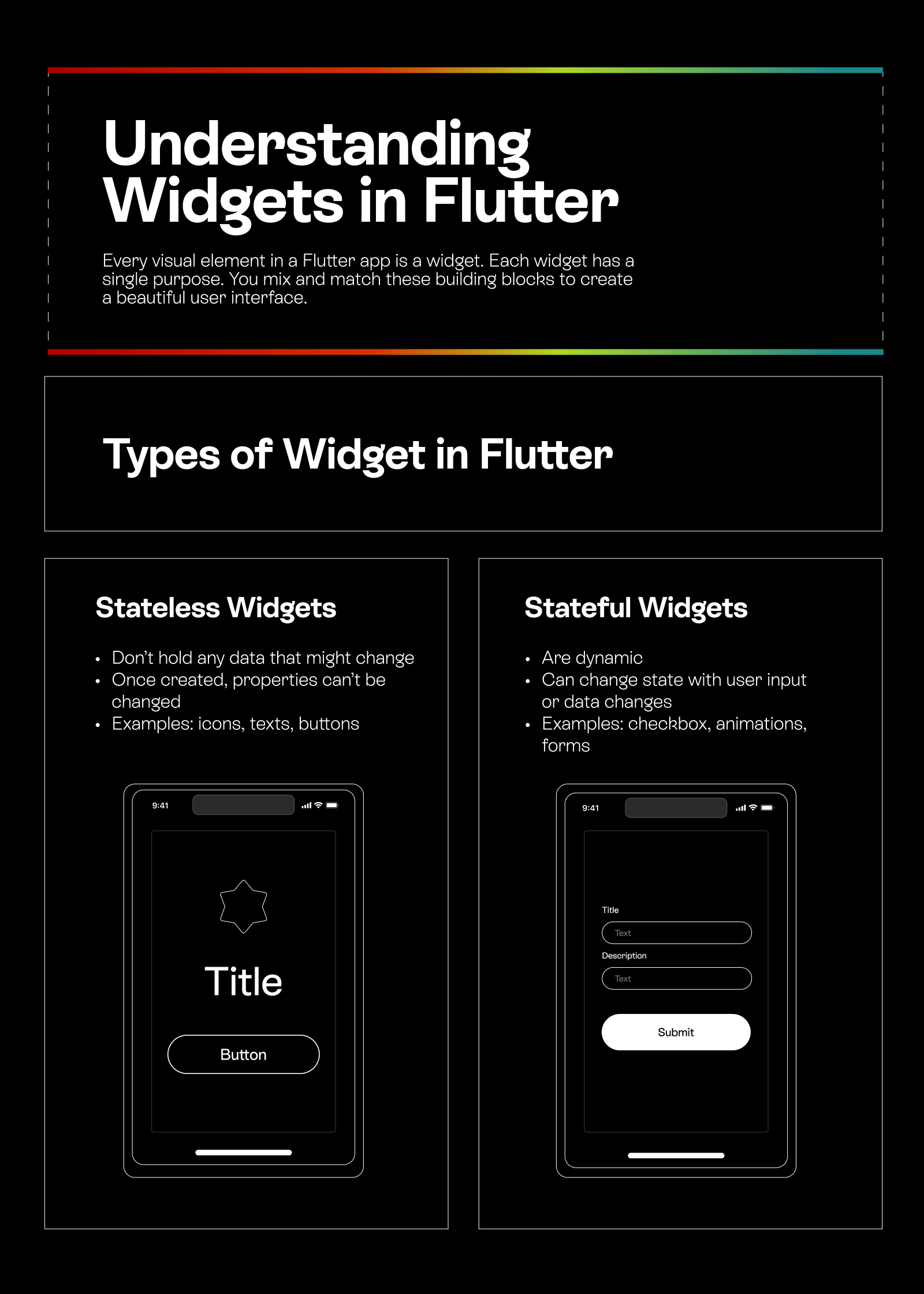
Flutter categorizes widgets into two main types: stateless widgets and stateful widgets.
Stateless Widgets
Stateless widgets don’t change their state during the lifetime of the widget. In simpler terms, they don’t hold any data that might change. Examples include icons, text, and buttons. Stateless widgets are immutable; once they’re created, their properties cannot change.
Stateful Widgets
Stateful widgets can change their state during the lifetime of the widget. They’re dynamic and can interact with user inputs or data changes. For example, a checkbox, an animation, or a form that changes when a user interacts with it are stateful widgets.
The Concept of 'Everything Is a Widget'
A core aspect of Flutter is that "everything is a widget". Everything you see in a Flutter app, from the layout structure itself (like rows and columns) to the text and images, is a widget, or a combination of widgets. This unified model simplifies the UI creation process, making it more intuitive and cohesive.
Widgets as the UI Constructors
Flutter widgets essentially construct the user interface. They describe what their view should look like with their current configuration and state. When a widget’s state changes, the widget rebuilds its description. The Flutter framework compares the new blueprint (the "diff") with the old one to see what specifically changed, determining the minimal changes needed in the underlying render tree.
In short, any updates in a widget's appearance triggers a process where only the necessary parts of the screen are refreshed—not the whole thing. This keeps your app responsive and performant.
The Core of Every Flutter UI
Creating UI Elements With Widgets
Every visual element in a Flutter app is a widget. From a simple text label (Text widget) to a button (FlatButton or ElevatedButton widget), widgets are used to create each part of the UI.
Certain widgets allow for detailed design implementations. For example, a Container widget offers customization for padding, margins, borders, and color.
Composing Complex UIs From Simple Widgets
Complex UI elements are built by composing simpler widgets together. For example, a login form can be created by combining text fields, buttons, and images.
This compositional approach promotes reusability and modularity. You can build custom widgets by combining existing ones, and reuse them across the app.
Layout Widgets
Layouts are handled by specific widgets like Row, Column, and Stack, which define how child widgets (or a widget nested inside another widget) are positioned and aligned.
Row and Column are used for linear horizontal and vertical layouts, respectively, while Stack allows for overlapping widgets.
Responsive Design
Widgets play a key role in creating responsive designs. Flutter provides widgets like MediaQuery, Flexible, and Expanded that adapt to different screen sizes and orientations.
Widget Properties and Nesting
Widgets are configured using their properties—these define the widget's appearance and behavior. For instance, a Text widget has properties like style, textAlign, and textDirection.
Widgets are often nested within one another to build the UI. This creates a hierarchy, known as the widget tree, which is key to Flutter's rendering process.
Example: Building a Simple UI Layout
Consider a simple UI with a Title, an Image, and a Button. This can be composed using a Column widget (a Layout widget) containing a Text widget for the title, an Image widget, and a FlatButton for the button.
Each widget is customized using its properties. Their arrangement is defined by the Column widget, demonstrating how various widgets interact to form a complete UI.
Understanding the Widget Tree
In Flutter, the concept of the widget tree is central to how applications are structured and rendered. We explore the widget tree's role in organizing and managing widgets in a Flutter app.
What Is a Widget Tree?
The widget tree is a hierarchical representation of widgets in a Flutter app. A widget tree tells you:
- The parent-child relationships between widgets. Some widgets, like a Row or Column, can hold other widgets inside them. These are called parent widgets, and the widgets they hold are child widgets.
- The order of the widgets. The order matters because it determines how things appear on the screen.
- The properties of each widget. Properties are like settings that define how a widget looks and behaves, like the font size of a text label or the color of a button.
Widget Nesting and Hierarchy
Widgets are nested within each other, creating a hierarchy. This nesting determines the layout and order of rendering.
The parent widget often controls the layout and appearance of its child widgets. For example, a Column widget dictates the vertical arrangement of its children.
Understanding Rendering With the Widget Tree
Flutter uses the widget tree to determine how to draw the UI. It tells the framework which widgets to draw and how to put them together, making sure your app's UI appears exactly as you designed it.
When a widget’s state changes, the affected part of the tree is rebuilt, allowing for dynamic and responsive UIs.
What Is a Widget’s Context in Flutter?
In Flutter, each widget in the tree has a context, which refers to its position in the tree. Context is crucial for accessing data and other widgets in the tree.
Widget Tree in Action: An Example
Consider a simple app with a Scaffold containing an AppBar and a Body. The Scaffold is the root of this part of the widget tree, with AppBar and Body as its children.
Inside the Body, there could be a Column with various widgets like Text, Image, and Button. This further extends the tree, showing how widgets are nested and organized.
State Management in Widgets
Ever wondered how Flutter apps react to user taps and data updates? It's all thanks to state management. This section dives into state management in both stateless and stateful widgets, plus the role of widget composition in building dynamic and responsive Flutter apps.
State in Flutter: A Core Concept
Stateless Widgets and Stateful Widgets
Stateless Widgets are immutable, meaning they don’t change their state during their lifetime. They’re ideal for parts of the UI that are static and don’t require user interaction or data changes.
Examples include text labels, icons, and images. For example, the Text widget displays a string of text that doesn’t change unless the parent widget provides new data.
Stateful Widgets are designed to be dynamic. They can change their state during their lifetime. This is key for interactive elements like forms, animations, or any part of the UI that changes in response to user interactions or data updates.
A Stateful Widget is made of two classes: the Widget class itself and a State class. The State class holds the state data and logic, while the Widget class is responsible for constructing the State class.
Managing State Changes in Stateful Widgets
In Stateful Widgets, when the state changes (e.g., a user types into a form field), the widget rebuilds, updating the UI to reflect the new state.
Flutter provides various ways to trigger a rebuild, such as calling setState() in the State class, which signals the framework to redraw the widget with the updated state.
Widget Composition and State Management
- Local vs. Global State: State can be local (managed within a single widget) or global (shared across multiple parts of the app). Widget composition plays a crucial role in determining how state is managed and accessed.
- Passing State Down the Tree: In Flutter, state is often passed down the widget tree through constructors. Parent widgets can pass data to child widgets, which then use this data to build their UI.
- Lifting State Up: Sometimes, it's beneficial to lift the state up to a common ancestor to manage shared state more effectively. This approach simplifies state management by centralizing it in a single location.
Advanced State Management Libraries
- BLoC (Business Logic Component): BLoC is a popular pattern that separates the business logic from the UI. It uses streams and sinks to manage state and events, promoting a more reactive and scalable approach to state management.
- Riverpod: Riverpod is a newer state management solution that offers enhanced flexibility and testability compared to Provider. It allows for better control over the state and dependencies, making it a robust choice for complex applications.
- Other Libraries: There are several other state management solutions available in Flutter, such as Redux, MobX, and Provider. Each comes with its own set of principles and best practices, catering to different needs and complexity levels.
Best Practices in Widget Composition:
Widget composition is an art in Flutter development, requiring a thoughtful approach to create efficient, maintainable, and scalable applications. This subsection offers practical tips on effective widget composition and discusses common pitfalls to avoid.
Effective Widget Composition: Tips and Techniques
Keep Widgets Small and Focused:
- Aim for widgets that do one thing and do it well. Smaller, focused widgets are easier to maintain, test, and reuse.
- This approach aligns with the Single Responsibility Principle, enhancing the overall code quality.
Leverage Existing Widgets:
- Before creating custom widgets, explore Flutter's extensive widget library. Often, the functionality you need is already available in existing widgets.
- Combining and customizing existing widgets can save time and reduce code complexity.
Optimize for Reusability:
- Design widgets with reusability in mind. Creating generic, configurable widgets can make them adaptable to different parts of your application.
- Parameters and callbacks can be used to customize widget behavior and appearance.
Mindful State Management:
- Carefully consider where to manage state. Avoid unnecessary stateful widgets if the state can be managed higher in the widget tree or using state management libraries.
- Overuse of stateful widgets can lead to performance issues and complex code.
Use Const Constructors Where Possible:
- Utilize const constructors for widgets that do not require rebuilding. This can improve performance by reducing the need for widget re-creation.
- For example, use const Text('Hello') instead of Text('Hello') when the text does not change.
Common Pitfalls in Widget Composition and How to Avoid Them
Over-Nesting Widgets:
- Avoid deeply nesting widgets as it can make the code harder to read and maintain.
- Use helper methods or separate widgets to break down complex widget trees.
Ignoring the Widget Lifecycle:
- Understand the lifecycle of a widget, especially for stateful widgets. Mismanagement of the lifecycle can lead to memory leaks and performance issues.
- Properly manage resources like controllers, listeners, and streams in the lifecycle methods.
Neglecting Performance Considerations:
- Be mindful of the performance impact of your widgets. Excessive use of animations, shadows, or complex layouts can affect app performance.
- Profile and optimize your widgets, especially those that are part of frequently used screens.
Inadequate Testing of Custom Widgets:
- Custom widgets should be thoroughly tested, both in isolation and as part of the UI.
- Implement widget tests to ensure they work as expected and handle edge cases.
Example: Building a Simple UI
To enhance our example of building a simple profile screen in Flutter, let's apply the principles of "Don't Repeat Yourself" (DRY) and modularity. This approach involves creating reusable widgets and breaking down the UI into more manageable, modular components.
Refactoring for DRY and Modular Design
1. Creating a Reusable Text Widget
- Start by creating a custom text widget for reuse. This will ensure consistency and reduce repetition.
- For example, a ProfileText widget can be used for the name and bio.
2. Building the Profile Header
- Create a ProfileHeader widget that includes the user’s profile picture, name, and bio.
- This widget can be reused in different parts of the app, or in different apps.
3. Implementing the Post List With a Builder
- Refactor the list of posts into its own widget, PostList, using ListView.builder for dynamic generation.
- This makes the list component reusable and keeps the main profile screen widget clean and focused.
4. Assembling the Profile Screen
- With these modular components, the main profile screen becomes more concise and easier to manage.
Master the Art of Widget Composition in Flutter to Build Beautiful, Efficient Applications
Widget composition isn’t just a Flutter feature—it underpins efficient, beautiful, and useful Flutter applications. The concepts, best practices, and examples outlined in this guide are designed to empower you to code like a pro, the Apexive way.
Key Takeaways
Widgets as Building Blocks: Widgets are the fundamental elements in Flutter, serving as the building blocks for crafting user interfaces. Understanding how to use them effectively is crucial for any Flutter developer.
The Widget Tree: The hierarchical nature of the widget tree is central to how Flutter renders UIs. It's a concept that underscores the importance of thoughtful widget composition and organization.
State Management: Whether using simple stateful/stateless widgets or advanced state management solutions like BLoC or Riverpod, managing state is a key aspect of Flutter development that directly ties into how widgets are composed and interact.
Best Practices: Embracing best practices in widget composition, such as keeping widgets small and focused, optimizing for reusability, and being mindful of performance, can significantly enhance the quality and maintainability of your Flutter applications.
Practical Application: The example of building a simple UI demonstrates the practical application of these concepts, showing you how a modular and DRY (Don't Repeat Yourself) approach can lead to efficient and scalable widget composition.
Final Thoughts
Widget composition in Flutter is both an art and a science. It requires a balance of creativity, technical understanding, and strategic thinking. As you continue your Flutter development journey, keep experimenting with different ways to compose widgets. Remember, each project is an opportunity to refine your skills and push the boundaries of what's possible with Flutter.
Whether you're building simple interfaces or complex applications, the principles of widget composition will always be at the heart of your work. Embrace these concepts, and you're well on your way to mastering Flutter development.